Last updated on September 22nd, 2024 at 06:19 am
Ever wondered what Carbon Footprint your webpage left behind?
Let’s first establish what a Carbon Footprint is.
In the words of Emich.edu
A carbon footprint is the total greenhouse gas (GHG) emissions caused directly and indirectly by an individual, organization, event or product.
The internet consumes a lot of electricity and the same electricity (at least a large part of it) is generated while leaving the carbon footprint by emitting carbon emissions.
All the websites worldwide are powered by data centres which emit C02 emissions as the webpages are downloaded, served & consumed.
To be environmentally friendly & ensure that we are helping the environment it is essential that we make sure that our sites don’t emit a large amount of C02 emissions which will hurt the environment.
To evaluate the C02 emissions that the web pages emit in terms of Grams & Litres. I have built a Python Script that will help you measure these metrics.
Here is the Link to the Google Colab Notebook – https://colab.research.google.com/drive/1HwXVjj9VFARO-ow5nxD2HpHVDLxxDy3Q?usp=sharing
Here are the Script blocks which you can execute in the Google Colab Notebook
Step 1 – Install the libraries
# Install required libraries
!pip install requests pandas
Step 2 – Specify the List of URLs you want to get the scores for
# List of URLs to analyze
urls = [
"https://www.decodedigitalmarket.com/wikipedia-api-python-topical-map-visualize/",
"https://www.decodedigitalmarket.com/bert-vector-embeddings-compare-between-webpages-competitor/",
"https://www.decodedigitalmarket.com/bert-vector-embeddings-compare-between-webpages-competitor/",
"https://www.decodedigitalmarket.com/visualize-mom-url-clicks-search-console-api-python/"
# Add more URLs as needed
]
Step 3 – Imports & calling the API
We will be utilizing the API from api.websitecarbon.com
import requests
import pandas as pd
import time
# Initialize a list to store the results
results = []
# Base API endpoint
api_base = "https://api.websitecarbon.com/site"
# Iterate over each URL in the list
for url in urls:
try:
# Prepare the request parameters
params = {'url': url}
# Make the GET request to the API
response = requests.get(api_base, params=params)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Extract the tested URL (handles redirects or modified URLs)
tested_url = data.get('url', url)
# Determine if the hosting is green
green = data.get('green', 'unknown')
# Extract CO2 emissions data
co2_data = data.get('statistics', {}).get('co2', {})
# Prefer 'grid' data; fallback to 'renewable' if 'grid' is unavailable
grid_co2 = co2_data.get('grid', {})
renewable_co2 = co2_data.get('renewable', {})
grams = grid_co2.get('grams') if grid_co2 else renewable_co2.get('grams', 'N/A')
litres = grid_co2.get('litres') if grid_co2 else renewable_co2.get('litres', 'N/A')
# Append the data to the results list
results.append({
'URL': tested_url,
'Green Hosting': green,
'Grams CO2': grams,
'Litres CO2': litres
})
else:
print(f"Failed to retrieve data for {url}. Status Code: {response.status_code}")
results.append({
'URL': url,
'Green Hosting': 'Error',
'Grams CO2': 'Error',
'Litres CO2': 'Error'
})
except Exception as e:
print(f"An error occurred for {url}: {e}")
results.append({
'URL': url,
'Green Hosting': 'Error',
'Grams CO2': 'Error',
'Litres CO2': 'Error'
})
# Optional: Pause between requests to respect API rate limits
time.sleep(1) # Adjust as needed based on API guidelines
# Create a pandas DataFrame from the results
df = pd.DataFrame(results)
# Display the DataFrame
df
After this step, you can see the data visualized in a data frame

Step 4 – Download the results in a CSV
# Define the CSV filename
csv_filename = 'website_carbon_emissions.csv'
# Save the DataFrame to a CSV file
df.to_csv(csv_filename, index=False)
# Download the CSV file
from google.colab import files
files.download(csv_filename)
And that’s it! With this, you will get the scores. I have tested 4 blog post URLs and all 4 of them are below 0.3 Grams.
How does the rating system work?
CO2 Emissions per Pageview
Rating | Grams CO2e per pageview |
---|---|
A+ | 0.095 |
A | 0.186 |
B | 0.341 |
C | 0.493 |
D | 0.656 |
E | 0.846 |
F | ≥ 0.847 |
Based on this rating system to achieve A+ Score, the web page can’t emit C02 grams more than 0.095
This is why my web page falls into the B Rating.
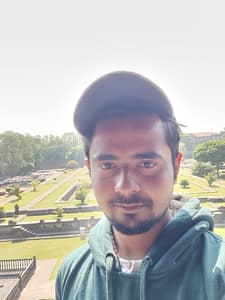
Kunjal Chawhan founder of Decode Digital Market, a Digital Marketer by profession, and a Digital Marketing Niche Blogger by passion, here to share my knowledge