In today’s post, I will talk about how you can build a text sentiment classification Google Sheets AppScript using Huggingface Inference API.
How will this help?
It will help you classify text on your Google Sheets cell into the following sentiments
- Positive
- Neutral
- Negative
AppScript will allow us to generate a formula using which we can effortlessly do the classification task.
Things we need:
- https://huggingface.co/cardiffnlp/twitter-roberta-base-sentiment-latest Model which I find to be pretty good as Sentiment Analysis.
- Huggingface Inference API (This guide explains how to get the API)
- AppScript
There is a limit to which you can use this API for free, I analyzed 800 cells for free just to set some context.
Why does it make sense to do Sentiment Classification on Google Sheets?
You may have PII (Personally Identifiable Information) in the Spreadsheet which you may not want to dump on ChatGPT Plus on Claude to conduct sentiment analysis otherwise you’re single-handedly responsible for leaking PII which you wouldn’t want.
Interesting Fact: I tried achieving this task via Python before but it wasn’t able to process long text cells for some reason on the spreadsheet I didn’t face this challenge.
Without further ado, here is the AppScript you Need to Paste in App Script
function SENTIMENT_ANALYSIS(input) {
// Hugging Face API endpoint
const API_URL = "https://api-inference.huggingface.co/models/cardiffnlp/twitter-roberta-base-sentiment-latest";
// Your Hugging Face API token
const API_TOKEN = "your_secret";
// Prepare the payload
const payload = {
inputs: input
};
// Set up the options for the API request
const options = {
method: "POST",
contentType: "application/json",
headers: {
"Authorization": "Bearer " + API_TOKEN
},
payload: JSON.stringify(payload)
};
try {
// Make the API request
const response = UrlFetchApp.fetch(API_URL, options);
const result = JSON.parse(response.getContentText());
// Process the result
if (result && result[0] && result[0].length > 0) {
const sentiments = result[0].map(item => ({ label: item.label, score: item.score }));
sentiments.sort((a, b) => b.score - a.score);
// Return the sentiment with the highest score
return sentiments[0].label;
} else {
return "Error: Unable to determine sentiment";
}
} catch (error) {
return "Error: " + error.toString();
}
}
This will generate SENTIMENT_ANALYSIS Function which can be used as a formula.

In Spreadsheet how to put the formula to get the classification done?
=SENTIMENT_ANALYSIS(A2)
If the text value is in cell A2, then this formula will classify that text in either of these buckets
- Positive
- Neutral
- Negative

Here is an example of how I got the sentiment classification done in the spreadsheet & believe me it took me just about 3-5 minutes to get it done.
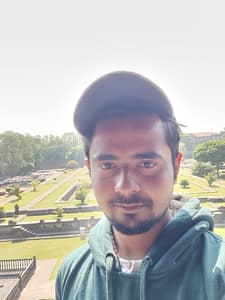
Kunjal Chawhan founder of Decode Digital Market, a Digital Marketer by profession, and a Digital Marketing Niche Blogger by passion, here to share my knowledge